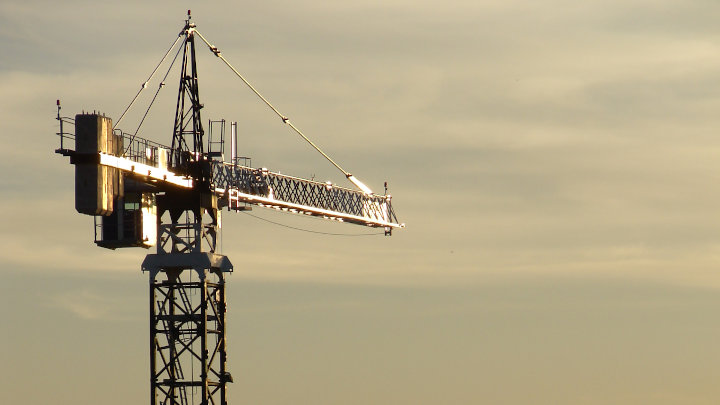
Object Serialization with JSON and compressed JSON in Python
22 Nov 2022Table of Contents
JSON is a popular data format for storing data in a structured way. Python has a built-in module called json that can be used to work with JSON data. In this article, we will see how to use the json module to serialize and deserialize data in Python.
Reading and Writing JSON in Python
Python offers out of the box a JSON encoder and decoder. To store and load JSON you can use the dump()
and load()
functions respectively. Since they are called the same as in pickling, this makes it easy to remember them.
import json
# Writing a JSON file
with open('data.json', 'w') as f:
json.dump(data, f)
# Reading a JSON file
with open('data.json', 'r') as f:
data = json.load(f)
You can additionally encode and decode JSON to a string which is done with the dumps()
and loads()
functions respectively. Encoding can be done like here:
json_string = json.dumps(data)
And to decode JSON you can type:
data = json.loads(json_string)
This comes handy when you work witk REST APIs where many APIs deal with JSON files as input and/or outputs.
Reading and Writing GZIP Compressed JSON in Python
It is also possible to compress the JSON in order to save storage space by typing the following:
import gzip
import json
with gzip.open("data.json.gz", 'wt', encoding='utf-8') as f:
json.dump(data, f)
To load the compressed JSON, type:
with gzip.open("data.json.gz", 'rt', encoding='utf-8') as f:
data = json.load(f)
This is especially useful when caching large amounts of JSON outputs.