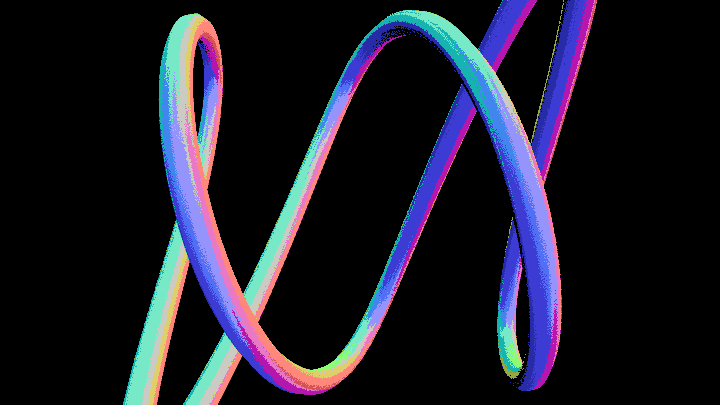
Videos and GIFs with Three.js
06 Apr 2017Table of Contents
Three.js is a powerful JavaScript library to create 3D computer graphics on the browser using WebGL. Here we’ll see how to create animations and videos from Three.js demos.
Prepare the Capture
For this task we will utilize CCapture.js, which is a handy library to capture frames from the canvas. It supports WebM, gifs or images in jpg or png collected in a tar file. Here we will focus on the image output of all frames because we want more control over the video and gif format.
Add the ccapture.js library in your project folder and add it to your html file
<script src="CCapture.all.min.js"></script>
To control the width and size of the frames simply set the size of the renderer.
const width = 600;
const height = 600;
const renderer = new THREE.WebGLRenderer({canvas: canvas, antialias: true});
renderer.setSize( width, height );
To start the capture in your JavaScript code just add the following code for png export
const capturer = new CCapture( { format: 'png' } );
capturer.start();
and to capture a frame, pass the canvas that you want to capture to the capturer
during the render loop
function render(){
requestAnimationFrame(render);
// render your scene
capturer.capture( canvas );
}
render();
After you have enough frames (e.g. specified number of frames) you can stop the capture and save the frames with
capturer.stop();
capturer.save();
Create GIF from Images
In our case we get a tar file which we need to extract (with e.g. 7zip and the command 7z x frames.tar
). Now we can create an mp4 video from the frames with ffmpeg by running the command
ffmpeg -i %07d.png video.mp4
There are further settings you can add to the command which can be found in the ffmpeg documentation. In order to create animated gifs from your frames you can use ImageMagick and Gifsicle. With ImageMagick you can create a gif animation by running the following command in the folder with the frames
convert -delay 4 -loop 0 *.png animation.gif
where the -delay
flag specifies the delay of each frame in ticks-per-second and the -loop
flag specifies the number of loops, which is with 0
an endless loop. Creating more efficient gifs is covered in this post.