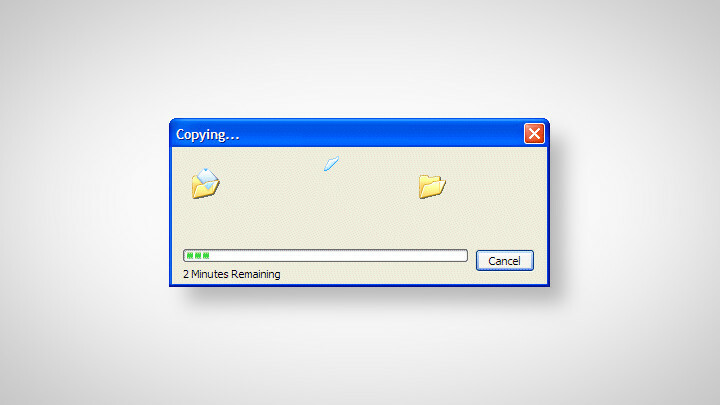
tqdm Cheat Sheet
20 Dec 2021Table of Contents
tqdm is a fast, user-friendly and extensible progress bar for Python and shell programs. Here you’ll find a collection of useful commands for quick reference.
Installation
Install tqdm with:
# With pip
pip install tqdm
# With anaconda
conda install -c conda-forge tqdm
To install tqdm for JupyterLab, you need to have ipywidgets installed. You can install it with:
# With pip
pip install ipywidgets
# With anaconda
conda install -c conda-forge ipywidgets
Enable ipywidgets for jupyter with:
jupyter nbextension enable --py widgetsnbextension
Cheat Sheet
To import tqdm to work both for notebooks and shell programs type:
from tqdm.auto import tqdm
Iterate over a range with:
for i in tqdm(range(100)):
# do something
Add description to the progress bar with:
for i in tqdm(range(100), desc="First loop"):
# do something
Iterate over a Pandas table with:
for idx, row in tqdm(df.iterrows(), total=len(df)):
# do something with that row
Add changing description to progress bar:
pbar = tqdm(range(100))
for i in pbar:
pbar.set_description(f"Element {i:03d}")
# do something
Show progress for nested loops:
for i in tqdm(range(10)):
for j in tqdm(range(100), leave=False):
# do something
The option leave=False
discards nested bars upon completion.
Resources
- tqdm.github.io
- Github - tqdm/tqdm