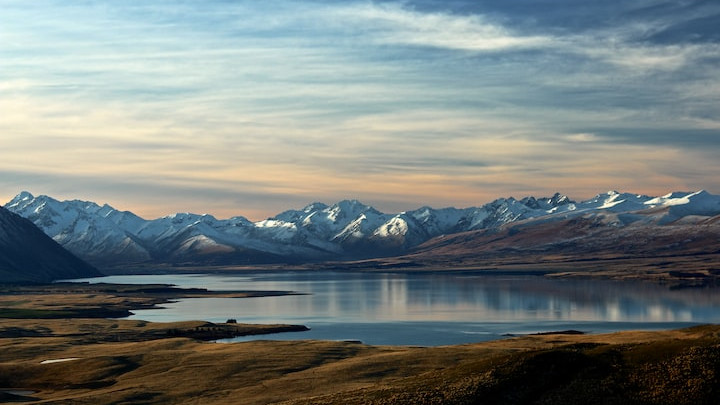
Downloading Images with Python, PIL, Requests, and Urllib
12 Apr 2023Table of Contents
- Download an Image with Requests
- Download an Image as PIL Image
- Download an Image using Urllib
- Resources
Python is a great language for automating tasks, and downloading images is one of those tasks that can be easily automated. In this article, you’ll see how to use the Python Imaging Library (PIL) or rather Pillow, Requests, and Urllib to download images from the web.
Download an Image with Requests
To get an image from a URL, you can use the requests package with the following lines of code. (Note, this was tested using requests 2.27.1
) In this case the script will download this picture from Lake Tekapo, New Zealand by Tobias Keller on Unsplash:
import requests
from PIL import Image
filepath = "assets/unsplash_image.jpg"
url = "https://images.unsplash.com/photo-1465056836041-7f43ac27dcb5?w=720"
r = requests.get(url)
if r.status_code == 200:
with open(filepath, 'wb') as f:
f.write(r.content)
Download an Image as PIL Image
In some cases, it is not necessary or possible to save the image somewhere and the image needs to be processed right away. In this case the whole image needs to be streamed by setting parameter stream=True
. For more information have a look at the documentation. Then the output needs to be converted into a io.BytesIO binary stream to be consumed by Pillow:
import io
import requests
from PIL import Image
w, h = 800, 600
filepath = "image.jpg"
url = "https://images.unsplash.com/photo-1465056836041-7f43ac27dcb5?w=720"
r = requests.get(url, stream=True)
if r.status_code == 200:
img = Image.open(io.BytesIO(r.content))
# Do something with image
# Save image to file
img.save(filepath)
Download an Image using Urllib
Sometimes you cannot install the requests library and need to use the rich Python standard library. In this case, you can use urllib.urlretrieve from the urllib package:
import urllib.request
w, h = 800, 600
filepath = "image.jpg"
url = "https://images.unsplash.com/photo-1465056836041-7f43ac27dcb5?w=720"
urllib.request.urlretrieve(url, filepath)