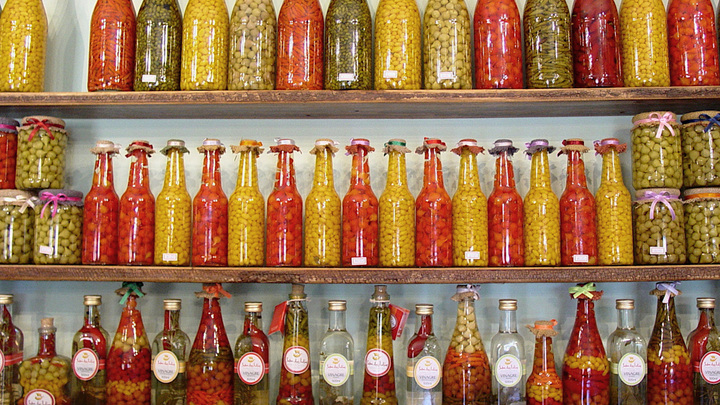
Object Serialization with Pickle in Python
28 Nov 2018Table of Contents
Python’s pickle module is a powerful tool for serializing and deserializing Python objects. Pickle can take almost any Python object and turn it into a string representation, which can then be stored in a file, database, or transmitted over a network connection.
Reading and Writing Pickle in Python
Pickle is used for serializing and de-serializing Python objects. This is a great way to store intermediate results while computing things. Pickling and unpickling can be done with the two functions dump()
and load()
respectively. The only thing you have to take care is that you open the file in binary mode. This is how you pickle your file:
import pickle
with open('data.pkl', 'wb') as f:
pickle.dump(data, f)
And this is how you unpickle your file:
with open('data.pkl', 'rb') as f:
data = pickle.load(f)
It is also possible to pickle an object to a bytes sequence with the dumps()
function:
bytes_sequence = pickle.dumps(data)
You can get the object back with the loads()
function:
data = pickle.loads(bytes_sequence)